SpotPlugin Class Reference
Plugin for the FROG system allowing the use of Sun Microsystem's SPOTs as a device. More...
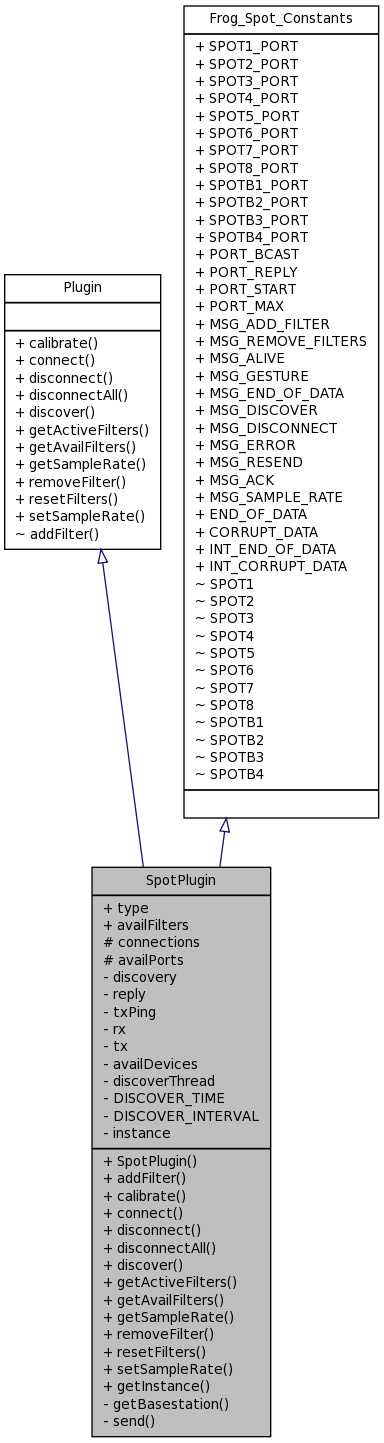
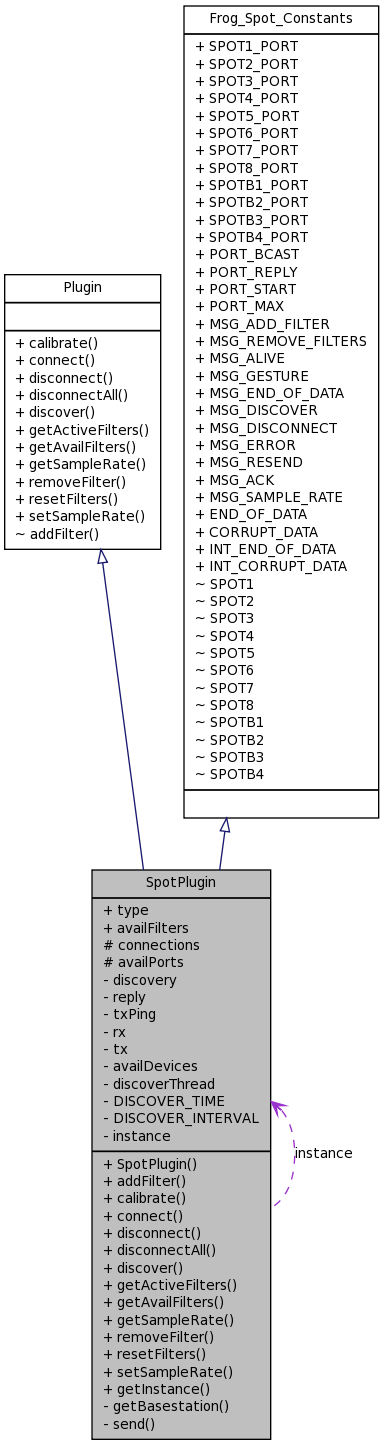
Public Member Functions | |
SpotPlugin () throws Throwable | |
Creates a single SpotPlugin object. | |
void | addFilter (Device device, int id, String arg) throws IOException |
Instruct the device to add a new filter to its filtering pipeline. | |
void | calibrate (Device device) throws IOException |
Calibrates the accelerometers of the target device. | |
synchronized void | connect (final Device device) throws IOException |
void | disconnect (Device device) throws IOException |
Disconnect a connected device. | |
void | disconnectAll () throws IOException |
Disconnect from all devices known to this Plugin. | |
void | discover (Vector< Device > deviceList) throws IOException |
A non-blocking method for finding nearby discoverable devices. | |
String[] | getActiveFilters (Device device) throws IOException |
Returns a list of filters currently active on the device. | |
String[] | getAvailFilters () |
Returns a list of available filters that this device supports. | |
int | getSampleRate (Device device) throws IOException |
Gets the current sampling rate of this Device in hertz. | |
void | removeFilter (Device device, int index) throws IOException |
Removes a filter from the list of active filters on the Device. | |
void | resetFilters (Device device) throws IOException |
Removes any filters currently active on the Device. | |
void | setSampleRate (Device device, int rate) throws IOException |
Instructs this device to sample at a given rate. | |
void | connect (Device device) throws IOException |
Formally connect to a specified device. | |
Static Public Member Functions | |
static SpotPlugin | getInstance () |
Retrieves the singleton object of SpotPlugin. | |
Static Public Attributes | |
static final String | type = "Sun SPOT" |
Applied to any Device object we create. | |
static final String[] | availFilters |
Filters that the SPOT is capable of performing. | |
static final String | SPOT1_PORT = "COM14" |
static final String | SPOT2_PORT = "COM9" |
static final String | SPOT3_PORT = "COM13" |
static final String | SPOT4_PORT = "COM15" |
static final String | SPOT5_PORT = "COM12" |
static final String | SPOT6_PORT = "COM10" |
static final String | SPOT7_PORT = "COM11" |
static final String | SPOT8_PORT = "COM8" |
static final String | SPOTB1_PORT = "COM4" |
static final String | SPOTB2_PORT = "COM7" |
static final String | SPOTB3_PORT = "COM6" |
static final String | SPOTB4_PORT = "COM5" |
static final short | PORT_BCAST = 32 |
static final short | PORT_REPLY = 33 |
static final short | PORT_START = 34 |
static final short | PORT_MAX = 254 |
static final byte | MSG_ADD_FILTER = -1 |
Messages sent between host and SPOT. | |
static final byte | MSG_REMOVE_FILTERS = -2 |
static final byte | MSG_ALIVE = -3 |
static final byte | MSG_GESTURE = -4 |
static final byte | MSG_END_OF_DATA = -5 |
static final byte | MSG_DISCOVER = -6 |
static final byte | MSG_DISCONNECT = -7 |
static final byte | MSG_ERROR = -8 |
static final byte | MSG_RESEND = -9 |
static final byte | MSG_ACK = -10 |
static final byte | MSG_SAMPLE_RATE = -11 |
static final double[] | END_OF_DATA = { 42.0 } |
Special markers for FilterPipeline on the SPOT. | |
static final double[] | CORRUPT_DATA = { 43.0 } |
static final int[] | INT_END_OF_DATA = { 42 } |
static final int[] | INT_CORRUPT_DATA = { 43 } |
Static Protected Attributes | |
static final Hashtable< String, SpotConnection > | connections = new Hashtable<String,SpotConnection>(4) |
SpotConnections are Sun SPOTs that are connected and have DeviceListeners attached to them. | |
static final HashSet< Short > | availPorts |
A HashSet allows constant time look-up and addition. | |
Static Package Attributes | |
static final String | SPOT1 = "0014.4F01.0000.1BE9" |
static final String | SPOT2 = "0014.4F01.0000.1E37" |
static final String | SPOT3 = "0014.4F01.0000.1E98" |
static final String | SPOT4 = "0014.4F01.0000.2AAE" |
static final String | SPOT5 = "0014.4F01.0000.3B61" |
static final String | SPOT6 = "0014.4F01.0000.3B7D" |
static final String | SPOT7 = "0014.4F01.0000.4118" |
static final String | SPOT8 = "0014.4F01.0000.4213" |
static final String | SPOTB1 = "0014.4F01.0000.2C06" |
static final String | SPOTB2 = "0014.4F01.0000.22F3" |
static final String | SPOTB3 = "0014.4F01.0000.3122" |
static final String | SPOTB4 = "0014.4F01.0000.415E" |
Detailed Description
Plugin for the FROG system allowing the use of Sun Microsystem's SPOTs as a device.
Please see http://brazos.cs.tcu.edu for more documentation.
- See also:
- SpotConnection
Constructor & Destructor Documentation
SpotPlugin | ( | ) | throws Throwable |
Creates a single SpotPlugin object.
Attempts to call this constructor more than once will generate an Exception.

Member Function Documentation
void addFilter | ( | Device | device, | |
int | id, | |||
String | arg | |||
) | throws IOException |
Instruct the device to add a new filter to its filtering pipeline.
If the device supports on-board filtering, this method should communicate both what type of filter to use as well as an argument string for that filter. The id should come from the getAvailFilters method (it is the index of the array of the desired filter).
- Parameters:
-
device the device to send the new filter information to id the filter desired. This should be the index from the array of filters. arg the argument String for this filter
- Exceptions:
-
IOException if any communication error occurs
Implements Plugin.
void calibrate | ( | Device | device | ) | throws IOException |
Calibrates the accelerometers of the target device.
If the device requires calibration or any adjustment before use, this method can be called to provide a custom GUI instructing the user on how to set up their device (such as setting it flat on a table or throwing it out a window).
- Parameters:
-
device the device to be calibrated
- Exceptions:
-
IOException if any communication error occurs
- See also:
- Device.calibrate()
Implements Plugin.
void connect | ( | Device | device | ) | throws IOException [inherited] |
Formally connect to a specified device.
After the user has been presented with a list of discovered devices, the one they pick must be connected to so that a formal connection (and probably a new Thread) can be established and they can begin using the device.
- Parameters:
-
device the device to connect to
- Exceptions:
-
IOException if any communication error occurs. It should be assumed that if this exception is thrown, the device is NOT connected.
- See also:
- Device.connect(DeviceListener)
Implemented in BluetoothPlugin.

synchronized void connect | ( | final Device | device | ) | throws IOException |

void disconnect | ( | Device | device | ) | throws IOException |
Disconnect a connected device.
This method should tear down any facilities that were set up to support the device. This should be called by the user when they wish to disconnect the device.
Unlike unexpected disconnects (disconnects resulting from battery or communication failure), the disconnect() method should not notify DeviceListeners of anything. A call to this method means the disconnect is deliberate and expected.
- Parameters:
-
device the device to disconnect from
- Exceptions:
-
IOException if the device cannot be sent a disconnect signal. Regardless, a best attempt must be made to tear down any local resources tied to this device.
- See also:
- Device.disconnect()
Implements Plugin.

void disconnectAll | ( | ) | throws IOException |
Disconnect from all devices known to this Plugin.
Convenience method for FROG. Most likely to be called when FROG is in the process of shutting down.
- Exceptions:
-
IOException if any of the connected devices could not be sent a disconnect message. Regardless, FROG is probably shutting down and any local resources should be freed.
Implements Plugin.
void discover | ( | Vector< Device > | deviceList | ) | throws IOException |
A non-blocking method for finding nearby discoverable devices.
Ideally this Plugin should broadcast a message looking for nearby devices. As devices are found, they should be placed on the deviceList. Implementing classes are encouraged to try and make the discovery of devices within 10 seconds. Devices that are found should not be connected to, but rather added to the given Vector<Device> so that the caller can view devices as they become available.
This method is expected to be non-blocking. That is, a Plugin should launch the discovery process in a separate Thread to allow other Plugins to perform their respective discoveries simultaneously.
- Exceptions:
-
IOException any catastrophic errors occur. Should ideally be thrown if the radio or other hardware is missing. Communication errors between the plugin and a device are not a sufficient cause to throw this exception.
Implements Plugin.
String [] getActiveFilters | ( | Device | device | ) | throws IOException |
Returns a list of filters currently active on the device.
If the Device does not support on-device filtering, a null
or empty array may be returned.
- Returns:
- the list of currently active filters on the device in the order in which they were instantiated
- Exceptions:
-
IOException if a list of active filters could not be obtained from the Device.
- See also:
- Device.getActiveFilters()
Implements Plugin.
String [] getAvailFilters | ( | ) |
Returns a list of available filters that this device supports.
The array of strings returned by this method should be in a specific order as the index of the array will be used to communicate with the device later.
- Returns:
- a list of Strings containing the names of the filters supported
- See also:
- Device.getAvailFilters()
Implements Plugin.
static SpotPlugin getInstance | ( | ) | [static] |
Retrieves the singleton object of SpotPlugin.
- Returns:
- SpotPlugin's singleton object

int getSampleRate | ( | Device | device | ) | throws IOException |
Gets the current sampling rate of this Device in hertz.
- Returns:
- the sampling rate of this device in hertz
- Exceptions:
-
IOException if the current sample rate could not be obtained from the Device
- See also:
- Device.getSampleRate()
Implements Plugin.

void removeFilter | ( | Device | device, | |
int | index | |||
) | throws IOException |
Removes a filter from the list of active filters on the Device.
This method does nothing at all if on-device filtering is not supported.
- Parameters:
-
device the Device to delete a filter from index the position on the Device's active filters list to remove
- Exceptions:
-
IOException if the filter on the Device could not be removed
Implements Plugin.
void resetFilters | ( | Device | device | ) | throws IOException |
Removes any filters currently active on the Device.
This method makes it possible for the user to edit or remove filters the Device is performing. This method does nothing if on-device filtering cannot be done by this type of Device.
- Parameters:
-
device the Device to clear the filters on
- Exceptions:
-
IOException if any communication error occurs
Implements Plugin.
void setSampleRate | ( | Device | device, | |
int | rate | |||
) | throws IOException |
Instructs this device to sample at a given rate.
If this device is incompatible with setting a different sampling rate, this method does nothing.
- Parameters:
-
rate the rate to sample at in hertz
- Exceptions:
-
IOException if any communication error occurs
Implements Plugin.

Member Data Documentation
final String [] availFilters [static] |
{ "Directorial Equivalence", "Idle State", }
Filters that the SPOT is capable of performing.
final HashSet<Short> availPorts [static, protected] |
new HashSet<Short>((PORT_MAX - PORT_START))
A HashSet allows constant time look-up and addition.
We want that because we only want one instance of each port to exist in the set.
HashMaps are not thread-safe so the methods here that use them are synchronized.
final Hashtable<String,SpotConnection> connections = new Hashtable<String,SpotConnection>(4) [static, protected] |
SpotConnections are Sun SPOTs that are connected and have DeviceListeners attached to them.
In the event they are disconnected, unexpectedly or not, they should be remove from this list. This Hashtable starts out with 4 slots as, at least initially, FROG's GUI only supports 4 connected devices.
final double [] CORRUPT_DATA = { 43.0 } [static, inherited] |
final double [] END_OF_DATA = { 42.0 } [static, inherited] |
Special markers for FilterPipeline on the SPOT.
final int [] INT_CORRUPT_DATA = { 43 } [static, inherited] |
final int [] INT_END_OF_DATA = { 42 } [static, inherited] |
static final byte MSG_ACK = -10 [static, inherited] |
static final byte MSG_ADD_FILTER = -1 [static, inherited] |
Messages sent between host and SPOT.
static final byte MSG_ALIVE = -3 [static, inherited] |
static final byte MSG_DISCONNECT = -7 [static, inherited] |
static final byte MSG_DISCOVER = -6 [static, inherited] |
static final byte MSG_END_OF_DATA = -5 [static, inherited] |
static final byte MSG_ERROR = -8 [static, inherited] |
static final byte MSG_GESTURE = -4 [static, inherited] |
static final byte MSG_REMOVE_FILTERS = -2 [static, inherited] |
static final byte MSG_RESEND = -9 [static, inherited] |
static final byte MSG_SAMPLE_RATE = -11 [static, inherited] |
static final short PORT_BCAST = 32 [static, inherited] |
static final short PORT_MAX = 254 [static, inherited] |
static final short PORT_REPLY = 33 [static, inherited] |
static final short PORT_START = 34 [static, inherited] |
static final String SPOT1 = "0014.4F01.0000.1BE9" [static, package, inherited] |
static final String SPOT1_PORT = "COM14" [static, inherited] |
static final String SPOT2 = "0014.4F01.0000.1E37" [static, package, inherited] |
static final String SPOT2_PORT = "COM9" [static, inherited] |
static final String SPOT3 = "0014.4F01.0000.1E98" [static, package, inherited] |
static final String SPOT3_PORT = "COM13" [static, inherited] |
static final String SPOT4 = "0014.4F01.0000.2AAE" [static, package, inherited] |
static final String SPOT4_PORT = "COM15" [static, inherited] |
static final String SPOT5 = "0014.4F01.0000.3B61" [static, package, inherited] |
static final String SPOT5_PORT = "COM12" [static, inherited] |
static final String SPOT6 = "0014.4F01.0000.3B7D" [static, package, inherited] |
static final String SPOT6_PORT = "COM10" [static, inherited] |
static final String SPOT7 = "0014.4F01.0000.4118" [static, package, inherited] |
static final String SPOT7_PORT = "COM11" [static, inherited] |
static final String SPOT8 = "0014.4F01.0000.4213" [static, package, inherited] |
static final String SPOT8_PORT = "COM8" [static, inherited] |
static final String SPOTB1 = "0014.4F01.0000.2C06" [static, package, inherited] |
static final String SPOTB1_PORT = "COM4" [static, inherited] |
static final String SPOTB2 = "0014.4F01.0000.22F3" [static, package, inherited] |
static final String SPOTB2_PORT = "COM7" [static, inherited] |
static final String SPOTB3 = "0014.4F01.0000.3122" [static, package, inherited] |
static final String SPOTB3_PORT = "COM6" [static, inherited] |
static final String SPOTB4 = "0014.4F01.0000.415E" [static, package, inherited] |
static final String SPOTB4_PORT = "COM5" [static, inherited] |
The documentation for this class was generated from the following file:
- /Users/dev/Documents/SVN brazos.cs.tcu.edu/trunk/FROG/src/frog/plugin/spot/SpotPlugin.java