Session Class Reference
Session is the FROG representation of a single user. More...

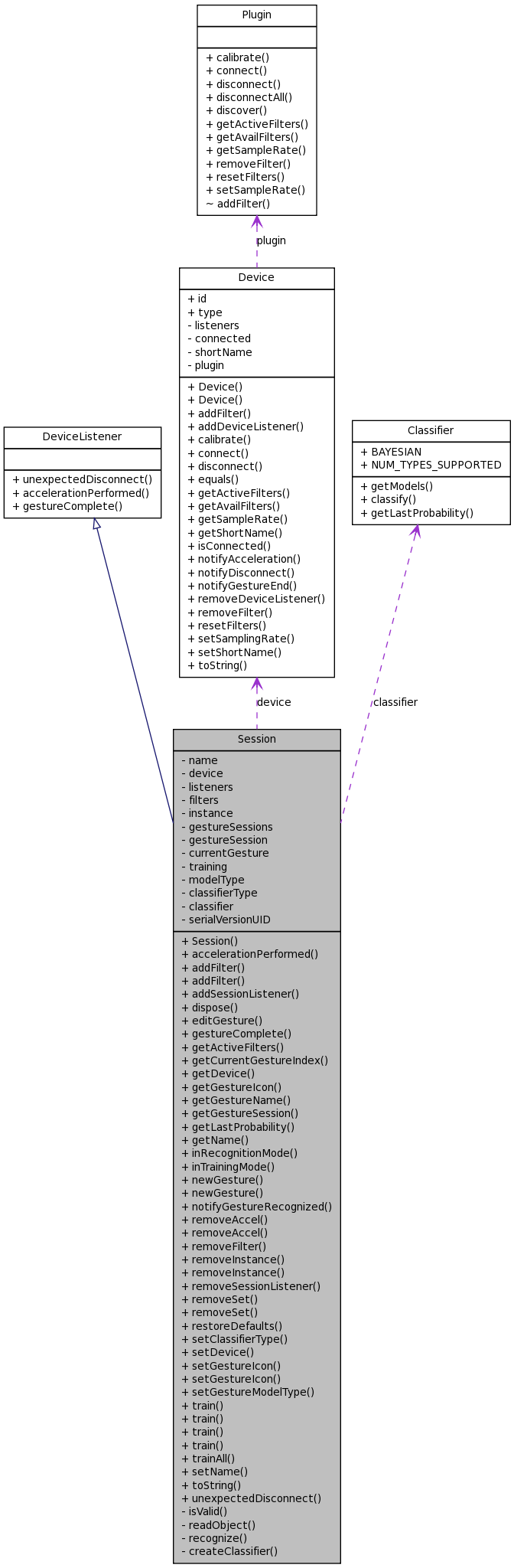
Public Member Functions | |
Session () | |
Creates a new Session. | |
void | accelerationPerformed (AccelEvent e) |
Run every time a single acceleration has come in. | |
void | addFilter (Filter filter) |
Adds a filter to this session. | |
void | addFilter (Filter filter, int index) |
Adds a filter in a particular order to this session. | |
void | addSessionListener (SessionListener sl) |
Adds a new SessionListener to this Session. | |
void | dispose () |
Completely destroys this session. | |
void | editGesture (int index) |
Sets the current gesture to the one specified by the index. | |
void | gestureComplete (boolean good, Device d) |
Run when a single gesture has been completed. | |
Filter[] | getActiveFilters () |
Returns a list of filters currently active on this Session. | |
int | getCurrentGestureIndex () |
Accessor for the current gesture index. | |
Device | getDevice () |
Returns the Device this Session is receiving acceleration data from. | |
BufferedImage | getGestureIcon (int index) |
Returns the icon of the gesture specified by the index. | |
String | getGestureName (int index) |
Returns the name of the gesture specified by the index. | |
Vector< GestureModel > | getGestureSession () |
Returns the gesture session stored by this Session object. | |
double | getLastProbability () |
Accessor for the last recognition event probability. | |
String | getName () |
Returns the name of this Session. | |
void | inRecognitionMode () |
Sets the mode to recognition, enabling/disabling certain functionality. | |
void | inTrainingMode () |
Sets the mode to training, enabling/disabling certain functionality. | |
GestureModel | newGesture (String name) |
Creates a new gesture and adds it to the current session. | |
GestureModel | newGesture (String name, byte[] imgBuf) throws IOException |
Creates a new gesture with a given image and adds it to the current session. | |
synchronized void | notifyGestureRecognized (GestureModel g) |
Notifies all SessionListeners of a recognition of a gesture g. | |
boolean | removeAccel (int inum, int anum) |
Deletes an individual acceleration from the current gesture in the current gesture session at the specified index (assuming the indices are valid). | |
boolean | removeAccel (int snum, int inum, int anum) |
Deletes an individual acceleration from the current gesture session at the specified index (assuming the indices are valid). | |
void | removeFilter (int i) |
Removes a filter at a given index. | |
boolean | removeInstance (int inum) |
Deletes a gesture instance from the current gesture in the current gesture session at the specified index (assuming the index is valid). | |
boolean | removeInstance (int snum, int inum) |
Deletes a gesture instance from current gesture session at the specified index (assuming the index is valid). | |
synchronized void | removeSessionListener (SessionListener sl) |
Removes a SessionListener from this Session's list of listeners. | |
boolean | removeSet () |
Deletes a gesture set from the current gesture session. | |
boolean | removeSet (int snum) |
Deletes a gesture set from the current gesture session at the specified index (assuming the index is valid). | |
void | restoreDefaults () |
Restores the default training parameters for this session. | |
void | setClassifierType (int type) |
Sets the type (an integer - see frog.Classifier) of classifier to be used in the Session. | |
void | setDevice (Device d) throws IOException |
Sets the device for this Session. | |
void | setGestureIcon (byte[] imageBuffer) throws IOException |
Sets the icon of the current gesture to the one contained in a byte array. | |
boolean | setGestureIcon (int index, byte[] imageBuffer) throws IOException |
Sets the icon of the specified gesture to the one contained in a byte array. | |
boolean | setGestureModelType (int type) |
Sets the type (an integer - see frog.GestureModel) of gesture model to be used in the Session. | |
boolean | train () |
Trains the GestureModel representing the current gesture in the current gesture session. | |
boolean | train (int index) |
Trains the GestureModel at the given index in the current gesture session. | |
boolean | train (int index, GestureHMMParameters gParams) |
Trains the GestureHMM at the given index in the current gesture session with the specified parameters. | |
boolean | train (int index, GestureHMMParameters gParams, KmeansParameters kParams) |
Trains the GestureHMM at the given index in the current gesture session with the specified parameters. | |
void | trainAll () |
Trains every GestureModel in the current gesture session. | |
void | setName (String name) |
Sets the name of this Session. | |
String | toString () |
void | unexpectedDisconnect (Device d) |
For handling an unexpected disconnect from a device. |
Detailed Description
Session is the FROG representation of a single user.
A session keeps track of all the objects and methods that must be run in a certain order on data passed to it from a device.
Constructor & Destructor Documentation
Member Function Documentation
void accelerationPerformed | ( | AccelEvent | e | ) |
Run every time a single acceleration has come in.
It is highly recommended you keep this method simple or execute in a new Thread. There may be many listeners all wanting to execute some code when an event happens.
- Parameters:
-
e contains the acceleration data and the calling device
Implements DeviceListener.

void addFilter | ( | Filter | filter, | |
int | index | |||
) |
Adds a filter in a particular order to this session.
- Parameters:
-
filter the filter to be added index the position in the filter order
- Exceptions:
-
ArrayOutOfBoundsException if the position was invalid
void addFilter | ( | Filter | filter | ) |
Adds a filter to this session.
- Parameters:
-
filter the filter to be added

void addSessionListener | ( | SessionListener | sl | ) |
Adds a new SessionListener to this Session.
- Parameters:
-
sl a new SessionListener to be notified of Session changes
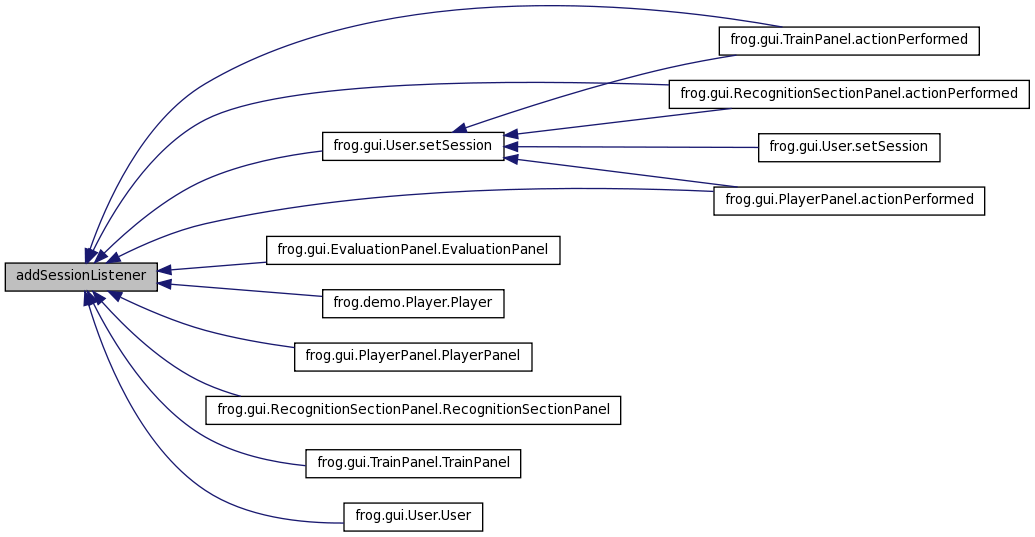
void dispose | ( | ) |
Completely destroys this session.
Readies it for garbage collection.

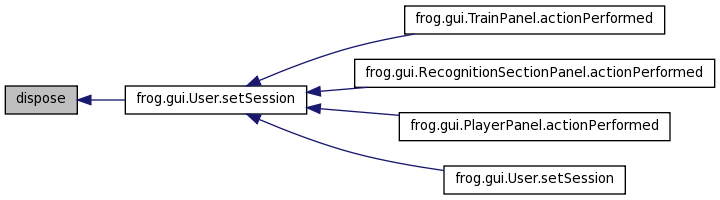
void editGesture | ( | int | index | ) |
Sets the current gesture to the one specified by the index.
This method does nothing if the index is invalid.
- Parameters:
-
index the index of the gesture to work on
void gestureComplete | ( | boolean | good, | |
Device | d | |||
) |
Run when a single gesture has been completed.
If the gesture was cut unexpectedly short either due to an error or hardware failure the boolean will be false and appropriate action should be taken to discard all acceleration data up to this point since the last known good gesture.
- Parameters:
-
good true
if this gesture is complete.false
if this gesture should be discarded.d the device that completed the gesture
Implements DeviceListener.
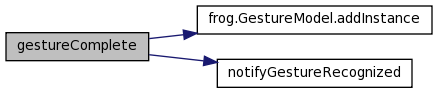
Filter [] getActiveFilters | ( | ) |
Returns a list of filters currently active on this Session.
- Returns:
- an array of all the Filters currently active in the correct order

int getCurrentGestureIndex | ( | ) |
Accessor for the current gesture index.
- Returns:
- the index of the current gesture

Device getDevice | ( | ) |
BufferedImage getGestureIcon | ( | int | index | ) |
Returns the icon of the gesture specified by the index.
This method returns null
if the GestureModel has no icon or if the index was invalid.
- Parameters:
-
index the index of the gesture to retrieve the icon of
- Returns:
- the icon of the gesture, or
null
if:- the gesture has no icon
- the index given was invalid

String getGestureName | ( | int | index | ) |
Returns the name of the gesture specified by the index.
If the index is invalid, this method returns null.
- Parameters:
-
index the index of the gesture to retrieve the name of
- Returns:
- the name of this gesture or
null
if the index was invalid


Vector<GestureModel> getGestureSession | ( | ) |
Returns the gesture session stored by this Session object.
The gesture session is all of the GestureModels combined. Each GestureModel represents one gesture, which itself is made up of several trainings of that gesture called gesture instances.
- Returns:
- the set of GestureModels currently loaded in the Session

double getLastProbability | ( | ) |
Accessor for the last recognition event probability.
This corresponds to the last probability obtained from the classifier associated with this session.
- Returns:
- the probability of the last recognition event


String getName | ( | ) |
void inRecognitionMode | ( | ) |
Sets the mode to recognition, enabling/disabling certain functionality.

void inTrainingMode | ( | ) |
Sets the mode to training, enabling/disabling certain functionality.
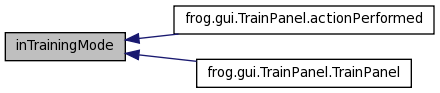
GestureModel newGesture | ( | String | name, | |
byte[] | imgBuf | |||
) | throws IOException |
Creates a new gesture with a given image and adds it to the current session.
- Parameters:
-
name the name to give this gesture; cannot be null
imgBuf the byte array of the image to use as an icon
- Returns:
- a reference to the new GestureModel for convenience. It has already been added to the Session.
- Exceptions:
-
IOException if there was a problem creating an icon from the given byte array
GestureModel newGesture | ( | String | name | ) |
Creates a new gesture and adds it to the current session.
- Parameters:
-
name the name to give this gesture; cannot be null
- Returns:
- a reference to the new GestureModel for convenience. It has already been added to the Session.
- Exceptions:
-
IllegalArgumentExecption if the gesture name is null
synchronized void notifyGestureRecognized | ( | GestureModel | g | ) |
Notifies all SessionListeners of a recognition of a gesture g.
- Parameters:
-
g the gesture g (a GestureModel) that was recognized

boolean removeAccel | ( | int | snum, | |
int | inum, | |||
int | anum | |||
) |
Deletes an individual acceleration from the current gesture session at the specified index (assuming the indices are valid).
- Parameters:
-
snum the index of the gesture set inum the index of the gesture instance anum the index of the Accel3D to delete
- Returns:
- a boolean that signifies the success or failure of deletion

boolean removeAccel | ( | int | inum, | |
int | anum | |||
) |
Deletes an individual acceleration from the current gesture in the current gesture session at the specified index (assuming the indices are valid).
- Parameters:
-
inum the index of the gesture instance anum the index of the Accel3D to delete
- Returns:
- signifies the success or failure of deletion

void removeFilter | ( | int | i | ) |
Removes a filter at a given index.
- Parameters:
-
i the index for deletion

boolean removeInstance | ( | int | snum, | |
int | inum | |||
) |
Deletes a gesture instance from current gesture session at the specified index (assuming the index is valid).
- Parameters:
-
snum the index of the gesture set inum the index of the instance to delete
- Returns:
- a boolean that signifies the success or failure of deletion

boolean removeInstance | ( | int | inum | ) |
Deletes a gesture instance from the current gesture in the current gesture session at the specified index (assuming the index is valid).
- Parameters:
-
inum the index of the instance to delete
- Returns:
- a boolean that signifies the success or failure of deletion


synchronized void removeSessionListener | ( | SessionListener | sl | ) |
Removes a SessionListener from this Session's list of listeners.
- Parameters:
-
sl The SessionListener to remove

boolean removeSet | ( | int | snum | ) |
Deletes a gesture set from the current gesture session at the specified index (assuming the index is valid).
- Parameters:
-
snum the index of the gesture set to delete
- Returns:
- a boolean that signifies the success or failure of deletion
boolean removeSet | ( | ) |
Deletes a gesture set from the current gesture session.
- Returns:
- a boolean that signifies the success or failure of deletion
void restoreDefaults | ( | ) |
Restores the default training parameters for this session.

void setClassifierType | ( | int | type | ) |
Sets the type (an integer - see frog.Classifier) of classifier to be used in the Session.
- Parameters:
-
type the classifier type
void setDevice | ( | Device | d | ) | throws IOException |
Sets the device for this Session.
Setting the Device causes the Session to attempt to connect to it if it isn't connected already. Note that the old Device is not disconnected first.
- Parameters:
-
d The device to connect to
- Exceptions:
-
IOException


boolean setGestureIcon | ( | int | index, | |
byte[] | imageBuffer | |||
) | throws IOException |
Sets the icon of the specified gesture to the one contained in a byte array.
- Parameters:
-
index the index of the gesture to modify imageBuffer the byte array containing the data to construct an image for the icon
- Returns:
true
if the index was valid;false
otherwise.
- Exceptions:
-
IOException if an image could not be constructed from the given byte array

void setGestureIcon | ( | byte[] | imageBuffer | ) | throws IOException |
Sets the icon of the current gesture to the one contained in a byte array.
- Parameters:
-
imageBuffer the byte array containing the data to construct an image for the icon
- Exceptions:
-
IOException if an image could not be constructed from the given byte array

boolean setGestureModelType | ( | int | type | ) |
Sets the type (an integer - see frog.GestureModel) of gesture model to be used in the Session.
This will modify which gesture session is currently in use. That is, Session can work on multiple different types of GestureModel sessions, but must focus on a single one at a time.
- Parameters:
-
type the gesture model type
- Returns:
- a boolean signifying success/failure (fails for an invalid type)
void setName | ( | String | name | ) |
String toString | ( | ) |

boolean train | ( | int | index, | |
GestureHMMParameters | gParams, | |||
KmeansParameters | kParams | |||
) |
Trains the GestureHMM at the given index in the current gesture session with the specified parameters.
If the current gesture session is not a GestureHMM session, an error will be reported. Training fails if the index is invalid.
Requires being in training mode.
- Parameters:
-
index gParams
- Returns:
- a boolean signifying success/failure of training

boolean train | ( | int | index, | |
GestureHMMParameters | gParams | |||
) |
Trains the GestureHMM at the given index in the current gesture session with the specified parameters.
If the current gesture session is not a GestureHMM session, an error will be reported. Training fails if the index is invalid.
Requires being in training mode.
- Parameters:
-
index the index of the GestureHMM to train gParams the parameters for the GestureHMM
- Returns:
- a boolean signifying success/failure of training

boolean train | ( | int | index | ) |
Trains the GestureModel at the given index in the current gesture session.
Training fails if the index is invalid. The type of GestureModel constructed depends on the current model type of the Session (as set by frog.Session#setGestureModelType(int).
Requires being in training mode.
- Parameters:
-
index the index of the GestureModel to train
- Returns:
- a boolean signifying success/failure of training

boolean train | ( | ) |
Trains the GestureModel representing the current gesture in the current gesture session.
The type of GestureModel constructed depends on the current model type of the Session (as set by frog.Session#setGestureModelType(int).
Requires being in training mode.
- Returns:
- a boolean signifying success/failure of training

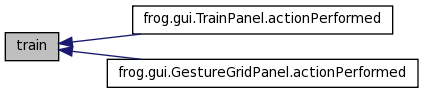
void trainAll | ( | ) |
Trains every GestureModel in the current gesture session.


void unexpectedDisconnect | ( | Device | d | ) |
For handling an unexpected disconnect from a device.
This is not called when a user has specified that they wish to disconnect. If a Device has gone out of range or the battery dies, that is an appropriate time to use this.
Common sense would dictate this is a job for Exceptions. However, if a disconnect occurs while idle, it is impossible to throw an exception beyond the Plugin. Devices need a listener to notify when something goes wrong.
- Parameters:
-
d Device that was unexpectedly disconnected
Implements DeviceListener.
The documentation for this class was generated from the following file:
- /Users/dev/Documents/SVN brazos.cs.tcu.edu/trunk/FROG/src/frog/Session.java